经过一段时间的JAVA实训学习,由以前的一窍不通,变成了今天的菜鸟,稍有成就感,o(∩_∩)o
下面就写一个小程序,把已经学过的知识都捎带着复习一下.. .. ..
先说程序的要求:小小的简单的学生信息管理系统。在控制台上显示四个选项,分别为增加、浏览、修改、退出系统;然后选择相应的功能进行操作。
先写主菜单吧,写上增加、浏览、修改和退出功能,这些功能当然不能写在这里,有下面的程序去实现。
main和menu:

main
package com.dr.demo.main;
import com.dr.demo.menu.Menu;
public class Main {
public static void main(String[] args) {
new Menu();
}
}
这就是程序的入口,超简洁。程序的所有代码都看不到,被封装在了后面。
menu:

menu
package com.dr.demo.menu;
import com.dr.demo.op.PersonOperate;
import com.dr.demo.util.InputData;
public class Menu {
InputData input = null;
public Menu(){
this.input = new InputData();
//循环出现菜单
while(true){
this.show();
}
}
//需要定义的菜单内容
public void show(){
System.out.println("\t\t\t1、增加人员信息");
System.out.println("\t\t\t2、浏览人员信息");
System.out.println("\t\t\t3、修改人员信息");
System.out.println("\t\t\t4、退出系统");
System.out.print("\n\n请选择要使用的操作:");
int temp = input.getInt();
switch(temp){
case 1:{ // 增加人员信息
new PersonOperate().add(); //业务处理层
break;
}
case 2:{ // 浏览人员信息
new PersonOperate().show();
break;
}
case 3:{ // 修改人员信息
new PersonOperate().update();
break;
}
case 4:{ //退出系统
System.out.println("选择的是退出系统");
System.out.println("系统退出!");
System.exit(1);
}
default:{
System.out.println("输入的内容不正确");
break;
}
}
}
}
开始是一段死循环的代码,让主菜单总是显示。
然后是switch--case,根据输入的内容执行相应的case。
退出系统就不用说了,三个功能分别由一下的代码实现:
增加,要知道增加的是什么,增加到什么地方(其他功能雷同)。Person类,然后便是这些功能的实现。
Person:

person
package com.dr.demo.vo;
import java.io.Serializable;
@SuppressWarnings("serial")
public class Person implements Serializable{
private String name;
private int age;
private float score;
public Person(){}
public Person(String name, int age, float score) {
this.name = name;
this.age = age;
this.score = score;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public float getScore() {
return score;
}
public void setScore(float score) {
this.score = score;
}
public String toString(){
return "姓名:"+this.name+",年龄:"+this.age+",成绩:"+this.score;
}
}
Person类实现Serializable接口(可序列化);设置Person的属性,实现set、get等方法。
PersonOperate,写出主程序中的功能的方法。

PersonOperate
package com.dr.demo.op;
import com.dr.demo.util.FileOperate;
import com.dr.demo.util.InputData;
import com.dr.demo.vo.Person;
public class PersonOperate {
private InputData input = null;
public PersonOperate(){
this.input = new InputData();
}
//完成具体的Person对象操作
public void add(){
//要使用输入数据的类
String name = null;
int age = 0;
float score = 0.0f;
System.out.print("输入姓名:");
name = this.input.getString();
System.out.print("输入年龄:");
age = this.input.getInt();
System.out.print("输入成绩:");
score = this.input.getFloat();
//生成Person对象,把对象保存在文件中
Person p = new Person(name,age,score);
try{
new FileOperate().save(p); //io操作层
System.out.println("数据保存成功!");
}catch(Exception e){
System.out.println("数据保存失败!");
}
}
public void show(){
//从文件中把内容读进来
Person p = null;
try{
p = (Person) new FileOperate().read();
}catch(Exception e){
System.out.println("内容显示失败,请确定数据是否存在!");
}
if(p != null){
System.out.println(p);
}
}
public void update(){
//先将之前的信息查出来
Person p = null;
try{
p = (Person) new FileOperate().read();
}catch(Exception e){
System.out.println("内容显示失败,请确定数据是否存在!");
}
if(p != null){
String name = null;
int age = 0;
float score =0.0f;
System.out.print("请输入新的姓名(原姓名:"+p.getName()+")");
name = this.input.getString();
System.out.print("请输入新的年龄(原年龄:"+p.getAge()+")");
age = this.input.getInt();
System.out.print("请输入新的成绩(原成绩:"+p.getScore()+")");
score = this.input.getFloat();
//信息重新设置
p.setName(name);
p.setAge(age);
p.setScore(score);
try{
new FileOperate().save(p);
System.out.println("数据更新成功!");
}catch(Exception e){
System.out.println("数据更新失败!");
}
}
}
}
这些数据都通过IO保存到文件中。add中的save,show中的read,update则read和save都用。
还有add和update都要识别输入数据,都要实现。
Input:

InputDate
package com.dr.demo.util;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class InputData {
private BufferedReader buf =null;
public InputData(){
buf = new BufferedReader(new InputStreamReader(System.in));
};
public String getString(){
String str = null;
try {
str = buf.readLine();
} catch (IOException e) {}
return str;
}
public int getInt(){
int temp = 0;
//如果输入的不是数字,至少应该有一个提示,告诉用户输入错了~
//可以使用正则验证
String str = null;
boolean flag = true;
while(flag){
//输入数据
str = this.getString();
if (!(str.matches("\\d+"))){
//如果输入的不是一个数字,则必须重新输入
System.out.print("输入的内容必须是整数,请重新输入:");
}else{
//输入的是一个正确的数字,则可以进行转换
temp = Integer.parseInt(str);
//表示退出循环
flag = false;
}
}
return temp;
}
public float getFloat(){
float f = 0.0f;
//如果输入的不是数字,至少应该有一个提示,告诉用户输入错了~
//可以使用正则验证
String str = null;
boolean flag = true;
while(flag){
//输入数据
str = this.getString();
if (!(str.matches("\\d+?.\\d{1,2}"))){
//如果输入的不是一个数字,则必须重新输入
System.out.print("输入的内容必须是小数(小数点后两位),请重新输入:");
}else{
//输入的是一个正确的数字,则可以进行转换
f = Float.parseFloat(str);
//表示退出循环
flag = false;
}
}
return f;
}
}
FileOperate:

FileOperate
package com.dr.demo.util;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import com.dr.demo.vo.Person;
public class FileOperate {
public static final String FILENAME = "E:\\person.ser";
//把对象保存在文件之中
public void save(Object obj){
ObjectOutputStream out = null;
try {
out = new ObjectOutputStream(new FileOutputStream(new File(FILENAME)));
//写入对象
out.writeObject(obj);
}catch(Exception e){
try {
throw e;
} catch (Exception e1) {}
}finally {
try {
out.close();
}catch(Exception e){}
}
}
//把对象从文件之中读出来
public Object read() throws Exception{
Object obj = null;
ObjectInputStream input =null;
try {
input = new ObjectInputStream(new FileInputStream(new File(FILENAME)));
obj = input.readObject();
} catch (Exception e) {
throw e;
}finally{
try{
input.close();
}catch(Exception e){}
}
return obj;
}
}
写完这些代码就可以实现增加、浏览、修改、退出系统的功能了。
运行结果:
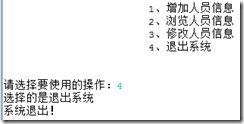
posted on 2010-11-06 22:12
Mineralwasser 阅读(204)
评论(0) 编辑 收藏